Collection Expressions in C# - Explained with examples
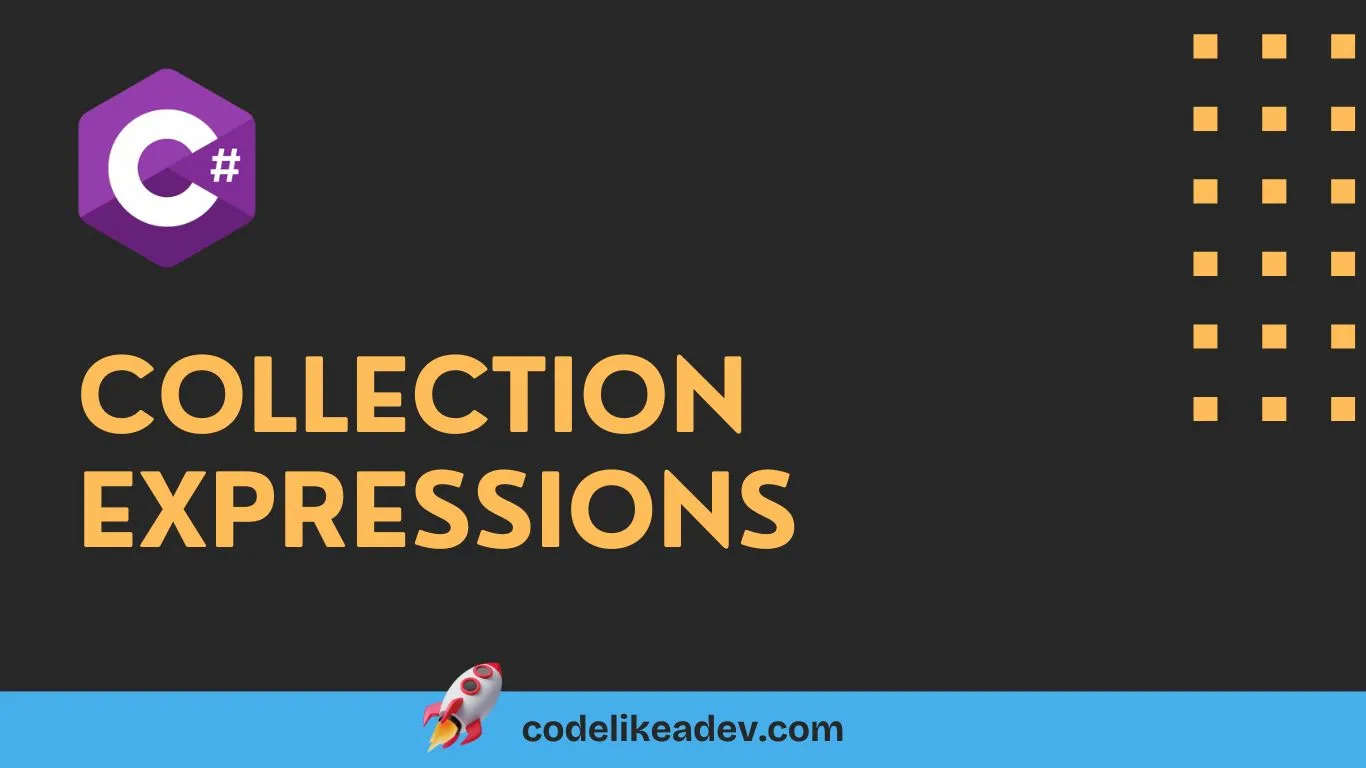
Collection expressions is a brand new feature introduced in C# 12. Collection expressions helps us to initialize and assign values to arrays, lists, spans and jagged arrays in a much easier way than the traditional way of assignment.
In this blog, we will explore what collection expressions are and how can we use them to initialize and assign values to arrays, lists and spans in C#.
A collection expression contains a sequence of elements within square brackets [ ].
Traditional way to initialize Arrays, Lists and Spans in C#
Before C# 12, there is no easy way to initialize arrays, lists and spans, you have to remember each type of initialization. Look at the examples below for the initialization for each type of Arrays, Lists and Spans.
We have to use the new
keyword to initialize and assign the values.
// Initialize Arrays
var arrayOfNumbers = new int[] { 1,2,3,4,5 };
// Initialize Lists
var listOfColors = new List<string> { "red", "green", "black" };
// Initialize Span
var arrayOfCharacters = new char[] { 'a', 'b', 'c', 'd', 'e' };
Span<char> spanOfCharacters = array3.AsSpan();
Changes to Initialization using Collection Expressions in C#
Now with C# 12, A _collection expressions can be converted to many different collection types. Values within the square brackets [] are evaluated and can be assigned to various collections like Arrays, Lists and Spans.
Here's how we would initialize Arrays, Lists and Spans using the new Collection expressions.
// Initialize Arrays
int[] arrayOfNumbers = [1,2,3,4,5];
// Initialize Lists
List<string> listOfColors = ["red", "green", "black"];
// Initialize Span
Span<char> spanOfCharacters = ['a', 'b', 'c', 'd', 'e'];
Using the collection expressions, you don't even have to use the new
keyword. The initialization of Arrays, Lists and Spans are the same and that makes our life soo easy.
I think I'll be using collection expressions from now on, the only reason is now I don't have to remember collection specific initialization.